Introduction to C++
C++ is a high-level programming language that serves as an extension of C, offering object-oriented features and other enhancements. It was developed by Bjarne Stroustrup and first appeared in 1985.
Features of C++
- Object-Oriented: Supports classes and objects, making it easier to manage and organize complex software.
- Compiled Language: Requires a compiler to translate the code into machine language before it can be executed.
- Strongly Typed: Enforces strict type checking, which helps in minimizing errors.
- Performance: Known for its efficiency and performance, making it suitable for system software, game development, and real-time simulations.
- Standard Template Library (STL): Provides a rich set of standard data structures and algorithms.
Basic Syntax:
#include < iostream >
using namespace std;
int main()
{
cout<<"Hellow to c++";
return 0;
}
Applications of C++
- Operating Systems: Many operating systems, including Microsoft Windows and Apple's OS X, have components that are written in C++ due to its performance and reliability.
- Game Development: C++ is extensively used in game development for its high performance. Popular game engines like Unreal Engine and game studios like EA and Ubisoft use C++.
- Embedded Systems: C++ is used in developing firmware for embedded systems due to its efficient handling of hardware resources.
- Financial Software: It's used in trading platforms and financial modeling software, where performance is crucial.
Online C++ Compiler
Introduction to Array
What is an Array?
An array is a linear data structure that consists of a collection of elements, all of the same data type, stored at contiguous memory locations 1 . This structure allows for efficient access to elements using an index, which is typically a non-negative integer. The position of each element can be computed from its index using a mathematical formula
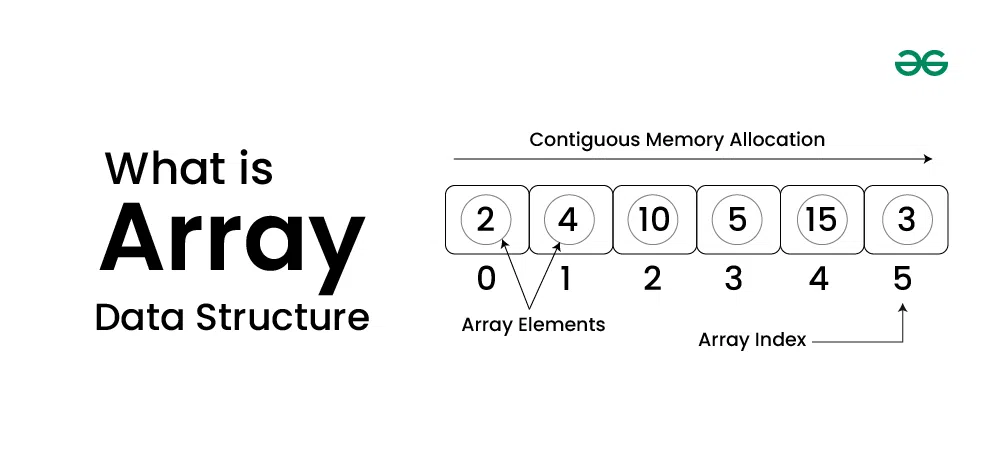
Charachterstics of Arrays
- Fixed size
- Indexed
- Homogenous element
Types of Arrays
- One dimensional
- Multi dimensional
- Dynamic dimensional
Tutorial for array
Introduction to Trees
What is a Tree?
A tree is a hierarchical data structure that consists of nodes connected by edges. It is used to represent and organize data in a way that is easy to navigate and search. The topmost node is called the root, and the nodes below it are called child nodes. Each node can have multiple child nodes, forming a recursive structure
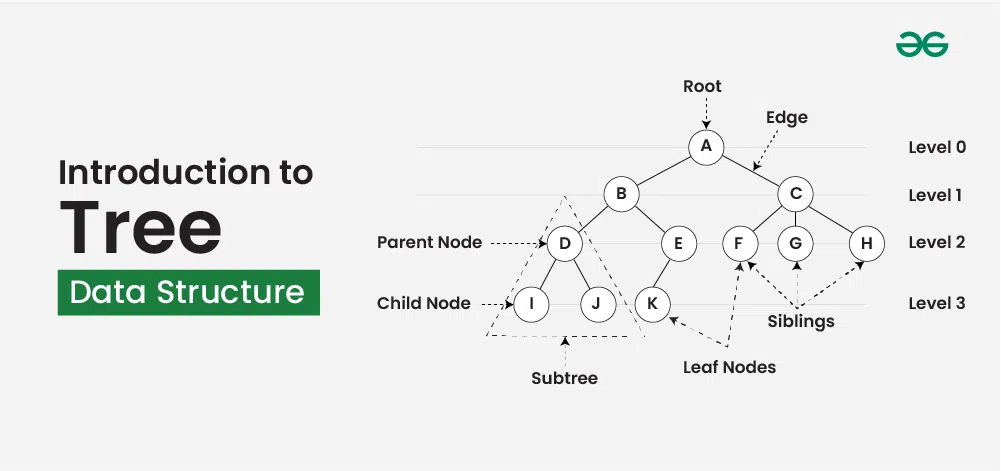
Charachterstics of trees
- Root Node: The topmost node of the tree, which does not have any parent node.
- Parent Node: A node that has one or more child nodes.
- Child Node: A node that is the immediate successor of another node.
- Leaf Node: A node that does not have any child nodes.
- Internal Node: A node with at least one child.
Types of Trees
- Binary Tree
- Ternary Tree
- N-ary Tree
Tutorial for Tree
Introduction to Graphs
What is a What is a Graph?
A graph is a non-linear data structure consisting of vertices (nodes) and edges that connect pairs of vertices. Graphs are used to represent relationships between different entities and are particularly useful in fields such as social network analysis, recommendation systems, and computer networks
Charachterstics of Graph
- Vertices (Nodes): The fundamental units of a graph which hold data. Each vertex can be connected to other vertices.
- Edges: Connections between vertices, representing the relationship between them. They can be directed or undirected.
- Degree of a Vertex: The number of edges connected to a vertex. In directed graphs, it is divided into in-degree (incoming edges) and out-degree (outgoing edges).
- Path: A sequence of edges that connect a sequence of vertices. A path can be simple (no repeated vertices) or may contain cycles.
- Cycle: A path that starts and ends at the same vertex, with no other repeated vertices in between.
Types of Graph
- Undirected Graph
- Directed Graph (DiGraph)
- Weighted Graph
- Unweighted Graph
- Cyclic Graph
- Acyclic Graph